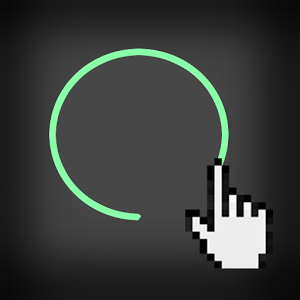
Steps
- Create New Application and give name it ImageSwitcher and Do next.
- minSdkVersion="17”
- targetSdkVersion="17".
- Give activity name – Main.java and main.xml.
- Go to main.xml and paste the below code.
Main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingTop="?attr/actionBarSize"> <ImageSwitcher android:layout_width="match_parent" android:layout_height="match_parent" android:id="@+id/imageSwitcher" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin"> <ImageView android:id="@+id/imageView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:scaleType="centerCrop" android:src="@mipmap/ic_launcher"/> </ImageSwitcher> </RelativeLayout>
Now your layout is set. Now Copy paste below Code in your Main.java file. in java file i have overridden onTouchEvent Method. that will accept parameter as event that user have done. In create method i have created cursor that will fetch images from SD card. some time it won't work. if you have same problem you have to add onfilling method.in image view i have set scale type as center crop that will stretch images from center and display but you can set different parameters in it .that will also accept Touch event as parameter and then you can do swipe operation.
Main.java
package com.example.samplecollection; import android.app.Activity; import android.database.Cursor; import android.net.Uri; import android.os.Bundle; import android.provider.MediaStore; import android.view.Menu; import android.view.MotionEvent; import android.widget.ImageSwitcher; import android.widget.ImageView; import android.widget.Toast; public class ViewFlipper_Demo extends Activity { ImageSwitcher Switch; ImageView images; float initialX; private Cursor cursor; private int columnIndex, position = 0; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.view_flipper); Switch = (ImageSwitcher) findViewById(R.id.imageSwitcher1); images = (ImageView) findViewById(R.id.imageView1); String[] projection = {MediaStore.Images.Thumbnails._ID}; cursor = managedQuery(MediaStore.Images.Thumbnails.EXTERNAL_CONTENT_URI, projection, null, null, MediaStore.Images.Thumbnails._ID + ""); columnIndex = cursor.getColumnIndexOrThrow(MediaStore.Images.Thumbnails._ID); } @Override public boolean onTouchEvent(MotionEvent event) { // TODO Auto-generated method stub switch (event.getAction()) { case MotionEvent.ACTION_DOWN: initialX = event.getX(); break; case MotionEvent.ACTION_UP: float finalX = event.getX(); if (initialX > finalX) { cursor.moveToPosition(position); int imageID = cursor.getInt(columnIndex); images.setImageURI(Uri.withAppendedPath(MediaStore.Images.Thumbnails.EXTERNAL_CONTENT_URI, "" + imageID)); //images.setBackgroundResource(R.drawable.mb__messagebar_divider); Switch.showNext(); Toast.makeText(getApplicationContext(), "Next Image", Toast.LENGTH_LONG).show(); position++; } else { if(position > 0) { cursor.moveToPosition(position); int imageID = cursor.getInt(columnIndex); images.setImageURI(Uri.withAppendedPath(MediaStore.Images.Thumbnails.EXTERNAL_CONTENT_URI, "" + imageID)); //images.setBackgroundResource(R.drawable.ic_launcher); Toast.makeText(getApplicationContext(), "previous Image", Toast.LENGTH_LONG).show(); Switch.showPrevious(); position= position-1; } else { Toast.makeText(getApplicationContext(), "No More Images To Swipe", Toast.LENGTH_LONG).show(); } } break; } return false; } }
Screen Shots
As you can see toast message will display when you swipe gesture on image switcher. copy paste all above code and change name as per your project. if you like this tutorial subscribe to my blog for future updates and best tutorials on android.
Keep Coding..
where is view_flipper__demo (in menu folder) code??
ReplyDeletePlease share it as soon as possible
Hey babu,
DeleteThat code is of no use so I removed it.
So now put this code and run it.